Developer Guide
- Acknowledgements
- Setting up, getting started
- Design
- Implementation
- Documentation, logging, testing, configuration, dev-ops
- Appendix: Requirements
- Appendix: Instructions for manual testing
- Appendix: Effort
- Appendix: Planned Enhancements
Acknowledgements
- This project is based on the AddressBook-Level3 project created by the SE-EDU initiative.
- The
remark
command was implemented with reference to the tutorial of adding a command.
Setting up, getting started
Refer to the guide Setting up and getting started.
Design

.puml
files used to create diagrams in this document docs/diagrams
folder. Refer to the PlantUML Tutorial at se-edu/guides to learn how to create and edit diagrams.
Architecture
The Architecture Diagram given above explains the high-level design of the App.
Given below is a quick overview of main components and how they interact with each other.
Main components of the architecture
Main
(consisting of classes Main
and MainApp
) is in charge of the app launch and shut down.
- At app launch, it initializes the other components in the correct sequence, and connects them up with each other.
- At shut down, it shuts down the other components and invokes cleanup methods where necessary.
The bulk of the app’s work is done by the following four components:
-
UI
: The UI of the App. -
Logic
: The command executor. -
Model
: Holds the data of the App in memory. -
Storage
: Reads data from, and writes data to, the hard disk.
Commons
represents a collection of classes used by multiple other components.
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete 1
.
Each of the four main components (also shown in the diagram above),
- defines its API in an
interface
with the same name as the Component. - implements its functionality using a concrete
{Component Name}Manager
class (which follows the corresponding APIinterface
mentioned in the previous point).
For example, the Logic
component defines its API in the Logic.java
interface and implements its functionality using the LogicManager.java
class which follows the Logic
interface. Other components interact with a given component through its interface rather than the concrete class (reason: to prevent outside component’s being coupled to the implementation of a component), as illustrated in the (partial) class diagram below.
The sections below give more details of each component.
UI component
The API of this component is specified in Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class which captures the commonalities between classes that represent parts of the visible GUI.
The UI
component uses the JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
- executes user commands using the
Logic
component. - listens for changes to
Model
data so that the UI can be updated with the modified data. - keeps a reference to the
Logic
component, because theUI
relies on theLogic
to execute commands. - depends on some classes in the
Model
component, as it displaysPerson
object residing in theModel
.
Logic component
API : Logic.java
Here’s a (partial) class diagram of the Logic
component:
The sequence diagram below illustrates the interactions within the Logic
component, taking execute("delete 1")
API call as an example.

DeleteCommandParser
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
How the Logic
component works:
- When
Logic
is called upon to execute a command, it is passed to anAddressBookParser
object which in turn creates a parser that matches the command (e.g.,DeleteCommandParser
) and uses it to parse the command. - This results in a
Command
object (more precisely, an object of one of its subclasses e.g.,DeleteCommand
) which is executed by theLogicManager
. - The command can communicate with the
Model
when it is executed (e.g. to delete a person). - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Here are the other classes in Logic
(omitted from the class diagram above) that are used for parsing a user command:
How the parsing works:
- When called upon to parse a user command, the
AddressBookParser
class creates anXYZCommandParser
(XYZ
is a placeholder for the specific command name e.g.,AddCommandParser
) which uses the other classes shown above to parse the user command and create aXYZCommand
object (e.g.,AddCommand
) which theAddressBookParser
returns back as aCommand
object. - All
XYZCommandParser
classes (e.g.,AddCommandParser
,DeleteCommandParser
, …) inherit from theParser
interface so that they can be treated similarly where possible e.g, during testing.
Model component
API : Model.java
The Model
component,
- stores the client list data i.e., all
Person
objects (which are contained in aUniquePersonList
object). - stores the currently ‘selected’
Person
objects (e.g., results of a search query) as a separate filtered list which is exposed to outsiders as an unmodifiableObservableList<Person>
that can be ‘observed’ e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. - stores a
UserPref
object that represents the user’s preferences. This is exposed to the outside as aReadOnlyUserPref
objects. - does not depend on any of the other three components (as the
Model
represents data entities of the domain, they should make sense on their own without depending on other components)
The Person
component,
- Has 6 compulsory attributes (
Name
,Phone
,Address
,Email
,Nric
,LicencePlate
) and 2 optional attributes (Remark
andTag
) -
Tag
can store any amount of tags - Has a
Policy
, which is optional for a person to have, but is stored in thePerson
class regardless- If a person has a policy attached to them,
Policy
will simply hold their information (i.e.Company
,PolicyNumber
, policy issue and expiry date asPolicyDate
) - If a person does not have a policy attached to them,
Policy
will hold default values
- If a person has a policy attached to them,

Tag
list in the AddressBook
, which Person
references. This allows AddressBook
to only require one Tag
object per unique tag, instead of each Person
needing their own Tag
objects.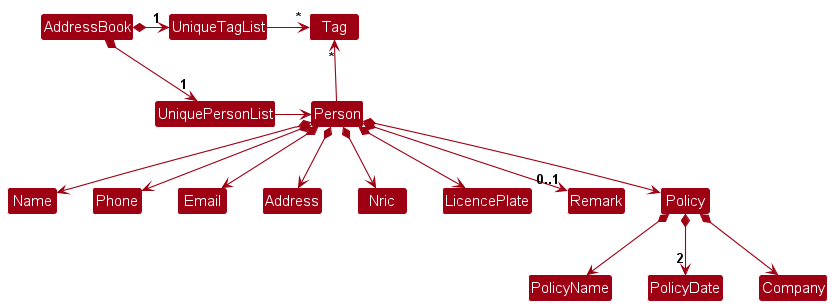
Design considerations
Aspect: Whether Policy
should be a class of its own
- Alternative 1: Add policy fields directly as attributes of
Person
- Pros: Less nested classes, easier implementation as AB3 already supports this style
- Cons: Hard to extend when more policy fields have to be added in the future (e.g.
PolicyType
)
- Alternative 2: (current choice) Abstract out
Policy
as its own class- Pros: A more OOP implementation and allows for easier extension
Aspect: How to handle clients with no policy
- Alternative 1: Make
Policy = null
forPerson
with no policy.- This was unsafe as when
Person
is displayed in the UI, methods liketoString()
would throw errors, violating type safety.
- This was unsafe as when
- Alternative 2: Make policy fields
Company
,PolicyNumber
andPolicyDate
benull
.- This was unsafe as the RegEx check (e.g.
isValidPolicyNumber()
) done in the constructors would have to be removed, leading to improper input validation.
- This was unsafe as the RegEx check (e.g.
- Alternative 3: (current choice) Have default policy parameters for policy fields.
- Though more checks have to be added when displaying the policies (which is a minor bug), it guarantees type safety and has input validation due to the more defensive programming approach taken
Storage component
API : Storage.java
The Storage
component,
- can save both client list data and user preference data in JSON format, and read them back into corresponding objects.
- inherits from both
AddressBookStorage
andUserPrefStorage
, which means it can be treated as either one (if only the functionality of only one is needed). - depends on some classes in the
Model
component (because theStorage
component’s job is to save/retrieve objects that belong to theModel
)
Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
Implementation
This section describes some noteworthy details on how certain features are implemented.
batchdelete
The batchdelete
command allows users to either batch delete people whose policy expiry date is in a specific month or
batch delete people whose policy was purchased from a specific company.
Implementation
In this section, we will use the case batch delete people whose policy expiry date is in a specific month to explain its implementation.
The batch delete mechanism is facilitated by DeleteMonth
.
It is also facilitated by the operation:
-
ModelManager#batchDeleteWithPredicate(Predicate<Person> predicate)
- batch delete all people in the client list who fulfil the given predicate.
This operation is exposed in the Model
interface as Model# batchDeleteWithPredicate(Predicate<Person> predicate)
.
The following sequence diagram shows how the batch delete operation works:
- instance of
DeleteMonth
is constructed to construct aBatchDeleteCommand
.

BatchDeleteCommand
and BatchDeleteCommandParser
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
- Calling method
updateFilteredPersonList
to get all people in the client list. - Using predicate p to get list of people to delete.
- Delete all people in the list and return all people left in client list.
Differences in the case batch delete people whose policy was purchased from a specific company:
- When constructing an instance of
BatchDeleteCommand
, an instance ofCompany
is passed as argument instead ofDeleteMonth
. - Construct an instance of
CompanyContainsKeywordsPredicate
instead ofPolicyExpiryInDeleteMonthPredicate
. - Predicate above is used when calling the method
batchDeleteWithPredicate
.
Design considerations
- Users may use batch delete to delete all people whose policy expiry is in the specified month and year. For example, delete people did not contact the user to renew their policies for one year.
- Besides, if users no longer work for an insurance company, they may like to delete people purchase policy from that company.
- Therefore,
Model#batchDeleteWithPredicate(Predicate<Person> predicate)
is introduced to allow batch delete by month or company by passing different predicates.
edit
The edit
command allows users to edit the details of a person in the client list.
Implementation
The edit mechanism is facilitated by EditCommand
. It extends Command
with an EditPersonDescriptor
to store the details of the person to be edited.
Additionally, it implements the following operations:
-
EditCommand#createEditedPerson(Person personToEdit, EditPersonDescriptor editPersonDescriptor)
— Creates and returns aPerson
with the details ofpersonToEdit
edited witheditPersonDescriptor
. -
EditCommand#execute(Model model)
— Edits the details of thePerson
in the client list.
The changes are finally made to the client list by calling Model#setPerson(Person target, Person editedPerson)
.
Given below is the sequence diagram for the edit
command:

EditCommandParser
and EditCommand
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
- Calling method setPerson to edit the person in client list.
- Calling method updateFilteredPersonList to update the client list with the edited person.
Design considerations
- Users may like to edit the details of a person in the client list, in case of changes in the personal details or policy of the person. For example, users may like to update the policy number of a person.
- Therefore,
EditCommand
is introduced to allow users to edit the details of a person in the client list.
remind
The remind
command allows the user to filter out people whose policy expiry date is approaching within the given number of days.
Implementation
The filtered list will be displayed in the UI. The remind mechanism is facilitated by Model
through the following operations:
-
Model#RemindPredicate(int days)
- The Predicate to be used for filtering.days
represents the number of days from the current date given by the user. -
Model#updateFilteredPersonList(Predicate<Person> p)
- Filters the list of Persons to display by the Predicatep
.
Given below is an example usage scenario and how the remind mechanism behaves at each step:
Step 1. The user realises that there may be a set of Persons whose policy expiry date is approaching within 30 days.
Step 2. The user executes remind 30
command in an attempt to find this set of Persons. A Model#RemindPredicate(int days)
instance with days
being 30
will be created. As described in the Logic Component above, this will create a RemindCommand
instance having the RemindPredicate
instance as its field.

Step 3. The LogicManager
will call RemindCommand#execute()
to start filtering the Persons list with the given RemindPredicate
. Then, Model#updateFilteredPersonList(Predicate<Person> p)
is called with the RemindPredicate
as the input to perform the filtering of the list.
Step 4. Finally, a CommandResult
instance will be created and returned to display filtered list of Persons to the user.
The following sequence diagram shows how the remind
command works:

RemindCommandParser
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram. The following activity diagram summarises what happens when a user executes the command remind 30
:
Design considerations
Aspect: Whether remind
command should take in a value:
- Alternative 1:
remind
command without specifying the number of days, default 30 days (which is 1 month).- Pros: Shorter command for the user to use, simply just one word.
- Cons: Not enough flexibility, user may want to find expiry dates beyond 30 days.
- Alternative 2 (current choice):
remind
command with number of days given by the user.- Pros: Allows more flexibility, now the user can find persons whose expiry dates is not only 30 days.
- Cons: Need to determine the range of days allowed for the user to enter, security concerns such as integer overflow could occur if user decides to perform malicious activities.
sort
The sort
command allows the user to view the profiles arranged in order of earliest to latest policy expiry date, with those profiles that have no policy data placed at the end of the late
Implementation
The sorted list will be displayed in the UI. The remind mechanism is facilitated by Model
through the following operations:
-
Model#SortData()
- The Unique Person List of the client list is sorted using aPolicyExpirationDateComparator
that implementsComparator<Person>
Given below is an example usage scenario and how the sort mechanism behaves at each step:
Step 1. The user wishes to see whose policy expires soon.
Step 2. The user executes sort
command to determine the persons whose insurance is going to finish the soonest. The Main Window
will call LogicManager#execute(String s)
. The LogicManager
in turn calls AddressBookParser#parseCommand(String s)
. Here the input is matched to SortCommandParser
and ParseCommand#execute()
is called.
Step 3. SortCommandParser
creates an instance of SortCommand
that is returned to LogicManager#execute(String) s
. The method CommandResult#execute()
is then called where Model#SortData()
is called.

sort
command, for instance sort 2
, no error will be thrown and the sorted list will be shown as normal. This is because the implementation ignores the arguments.
Step 4. Finally, a CommandResult
instance will be created and returned to display sorted list of Persons to the user.
The following sequence diagram shows how the sort
command works:
Design considerations
Aspect: Whether sort
command should take in a value.
- Alternative 1 (current choice):
sort
command does not take in a value but does not throw an exception if an input is produced.- Pros: Prevents the need for un necessary exceptions that might affect the running of the program
- Cons: The arguments might be nonsensical, for instance
sort 2
could instead be used to provide the 2 most closely expiring profiles.
- Alternative 2 :
sort
command does not take in a value and produces exception if an input is produced- Pros: Prevents nonsensical inputs
- Cons: Lack of functionality as specified for alternative 1. There is also a lack of flexibility.
- Alternative 3 :
sort
command takes in a value so the user can specify how many profiles they wish to view- Pros: Provides maximum flexibility and functionality
remark
The remark
command allows the user to add optional remarks to a person.
Implementation
This was implemented according to the tutorial of adding a command.
remark
works similarly to edit
, but is only editing the Remark
attribute of the Person
.
To add this feature, the following were done:
- Include optional
Remark
attribute inPerson
class - Add parsers to handle the
remark
command, with the functionality specified in the user guide
Design considerations
Aspect: Whether the prefix r/
is necessary.
- Alternative (not taken): To remove the need for the prefix such that the command format is
remark INDEX REMARK
- Pros: For a fast typer, this would save time to not need to type special characters like
/
- Cons: May lead to invalid behaviour not being flagged out appropriately, such as if user type
remark 1 2 Likes hiking!
, thinking it would add remarks to clients 1 and 2. - Cons: Does not leverage on existing
ArgumentMultimap
as a separate parsing of input is necessary, which gives way to more potential errors.
- Pros: For a fast typer, this would save time to not need to type special characters like
[Proposed] Undo/redo feature
Proposed Implementation
The proposed undo/redo mechanism is facilitated by VersionedAddressBook
. It extends AddressBook
with an undo/redo history, stored internally as an addressBookStateList
and currentStatePointer
. Additionally, it implements the following operations:
-
VersionedAddressBook#commit()
— Saves the current client list state in its history. -
VersionedAddressBook#undo()
— Restores the previous client list state from its history. -
VersionedAddressBook#redo()
— Restores a previously undone client list state from its history.
These operations are exposed in the Model
interface as Model#commitAddressBook()
, Model#undoAddressBook()
and Model#redoAddressBook()
respectively.
Given below is an example usage scenario and how the undo/redo mechanism behaves at each step.
Step 1. The user launches the application for the first time. The VersionedAddressBook
will be initialized with the initial client list state, and the currentStatePointer
pointing to that single client list state.
Step 2. The user executes delete 5
command to delete the 5th person in the client list. The delete
command calls Model#commitAddressBook()
, causing the modified state of the client list after the delete 5
command executes to be saved in the addressBookStateList
, and the currentStatePointer
is shifted to the newly inserted client list state.
Step 3. The user executes add n/David …
to add a new person. The add
command also calls Model#commitAddressBook()
, causing another modified client list state to be saved into the addressBookStateList
.

Model#commitAddressBook()
, so the client list state will not be saved into the addressBookStateList
.
Step 4. The user now decides that adding the person was a mistake, and decides to undo that action by executing the undo
command. The undo
command will call Model#undoAddressBook()
, which will shift the currentStatePointer
once to the left, pointing it to the previous client list state, and restores the client list to that state.

currentStatePointer
is at index 0, pointing to the initial state, then there are no previous states to restore. The undo
command uses Model#canUndoAddressBook()
to check if this is the case. If so, it will return an error to the user rather
than attempting to perform the undo.
The following sequence diagram shows how the undo operation works:

UndoCommand
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
The redo
command does the opposite — it calls Model#redoAddressBook()
, which shifts the currentStatePointer
once to the right, pointing to the previously undone state, and restores the client list to that state.

currentStatePointer
is at index addressBookStateList.size() - 1
, pointing to the latest client list state, then there are no undone states to restore. The redo
command uses Model#canRedoAddressBook()
to check if this is the case. If so, it will return an error to the user rather than attempting to perform the redo.
Step 5. The user then decides to execute the command list
. Commands that do not modify the client list, such as list
, will usually not call Model#commitAddressBook()
, Model#undoAddressBook()
or Model#redoAddressBook()
. Thus, the addressBookStateList
remains unchanged.
Step 6. The user executes clear
, which calls Model#commitAddressBook()
. Since the currentStatePointer
is not pointing at the end of the addressBookStateList
, all client list states after the currentStatePointer
will be purged. Reason: It no longer makes sense to redo the add n/David …
command. This is the behavior that most modern desktop applications follow.
The following activity diagram summarizes what happens when a user executes a new command:
Design considerations
Aspect: How undo & redo executes:
-
Alternative 1 (current choice): Saves the entire client list.
- Pros: Easy to implement.
- Cons: May have performance issues in terms of memory usage.
-
Alternative 2: Individual command knows how to undo/redo by
itself.
- Pros: Will use less memory (e.g. for
delete
, just save the person being deleted). - Cons: We must ensure that the implementation of each individual command are correct.
- Pros: Will use less memory (e.g. for
Documentation, logging, testing, configuration, dev-ops
Appendix: Requirements
Product scope
Target user profile:
- is a car insurance agent
- has a need to manage a significant number of contacts
- prefer desktop apps over other types
- can type fast
- prefers typing to mouse interactions
- is reasonably comfortable using CLI apps
Value proposition: Provide quick and easy contact management system for car insurance agents to keep track of multiple clients’ details and policies bought by them and have a better overview of the premium due dates.
User stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
* * * |
beginner user | add a client | expand my client list |
* * * |
beginner user | delete a client | remove entries who I am no longer working with |
* * * |
beginner user | find client(s) by fields | locate details of clients without having to go through the entire list |
* * * |
beginner user | edit a client | make changes to details of a client |
* * * |
expert user | export the InsureIQ data | have a secured copy of all my clients’ information |
* * |
first-time user | see the layout of the application filled with dummy data | learn how to use the application without corrupting my own data yet |
* * |
first-time user | delete all the dummy data | start using the application for my own use |
* * |
first-time user | import existing data into the system | have all my clients in one place for different insurance companies I am working for |
* * |
beginner-user | check which policies are close to completion | perform follow-up actions outside of the application |
* * |
experienced-user | have reminders for premium due dates expiry whenever I start the application | notify my clients about the upcoming payment |
* * |
experienced-user | add remarks to a client | better tailor my interactions with them |
* * |
expert user | sort clients by premium due date expiry | locate a client who needs to be urgently contacted |
* |
first-time user | have a tutorial function | familiarise myself with the features and understand how to use the application |
* |
experienced user | schedule follow-up calls or meetings with clients directly from the contact number in their profile | save the hassle of reaching out to them using another avenue |
* |
experienced user | review and categorise clients as leads or clients | prioritise my outreach efforts |
* |
experienced user | categorise clients by insurance company | easily find out which insurance company the client bought insurance from |
* |
experienced user | customise the layout of the application | better suit my preferences |
* |
expert user | generate reports on policy renewals and customer interactions | assess my performance |
* |
expert user | differentiate between polices based on coverage types | provide tailored advice to clients |
* |
expert user | track communication history with each client | provide personalised service |
* |
expert user | automatically edit a client who made their payment for the premium before the due date | save the hassle of editing the person manually |
* |
expert user | create custom commands | successfully perform tasks in a shorter time |
Use cases
(For all use cases below, the System is the InsureIQ
and the Actor is the User
, unless specified otherwise)
Use case: UC1 - List all clients
MSS
- User requests to list all clients.
-
InsureIQ shows a list of all clients.
Use case ends.
Use case: UC2 - Find clients
MSS
- User requests to list clients based on a specific condition.
-
InsureIQ shows a list of clients that satisfy the condition.
Use case ends.
Extensions
-
2a. The conditions specified are of invalid format.
-
2a1. InsureIQ shows an error message.
Use case ends.
-
Use case: UC3 - Add a client
MSS
- User requests to add a client.
-
InsureIQ shows a confirmation message that the client was added.
Use case ends.
Extensions
-
2a. The adding of the client fails.
-
2a1. InsureIQ shows an error message.
Use case ends.
-
Use case: UC4 - Delete a client
MSS
- User requests to list all clients (UC1) or find clients (UC2).
- InsureIQ shows a list of clients.
- User requests to delete a specific client in the list.
-
InsureIQ shows a confirmation message that the client was deleted.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. InsureIQ shows an error message.
Use case resumes at step 2.
-
Use case: UC5 - Edit a client
MSS
- User requests to list all clients (UC1) or find clients (UC2).
- InsureIQ shows a list of clients.
- User requests to edit a specific client in the list.
-
InsureIQ shows a confirmation message that the client was edited.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. InsureIQ shows an error message.
Use case resumes at step 2.
-
-
3b. The fields to be edited are of invalid format.
-
3b1. InsureIQ shows an error message.
Use case resumes at step 2.
-
Use case: UC6 – Modify remark of a client
MSS
- User requests to list all clients (UC1) or find clients (UC2).
- InsureIQ shows a list of clients.
- User requests to add or remove remark for a specific client in the list.
-
InsureIQ shows a confirmation message that the remark was modified.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. InsureIQ shows an error message.
Use case resumes at step 2.
-
Use case: UC7 – Get a reminder for clients with approaching policy expiry dates
MSS
- User requests to get a reminder for clients with approaching policy expiry dates within a certain number of days.
- InsureIQ filters the list based on the days given.
-
InsureIQ shows a confirmation message.
Use case ends.
Extensions
-
1a. The given number of days is invalid.
-
1a1. InsureIQ shows an error message.
Use case ends.
-
Use case: UC8 – Sort list of clients
MSS
- User requests to sort clients by policy expiry date.
- InsureIQ sorts the list of clients in the data file from earliest to latest policy expiry date.
-
InsureIQ shows a confirmation message that the list was sorted.
Use case ends.
Extensions
-
1a. There are no clients in the data file.
Use case ends.
Use case: UC9 – Batch delete clients
MSS
- User requests to batch delete clients, either by the policy company or by the month of the policy expiry date.
- InsureIQ delete all the clients who fulfil the specific condition in the data file.
-
InsureIQ shows a confirmation message that the specific clients were deleted.
Use case ends.
Extensions
-
1a. The conditions specified are of invalid format.
-
1a1. InsureIQ shows an error message.
Use case ends.
-
Use case: UC10 - Clear all clients
MSS
- User requests to clear all clients.
- InsureIQ clears all clients in the data file.
-
InsureIQ shows a confirmation message that all clients were cleared.
Use case ends.
Extensions
-
1a. There are no clients in the data file.
Use case ends.
Non-Functional Requirements
Should work on any mainstream OS as long as it has Java 11
or above installed.
-
Performance Requirements:
- The system should respond to user input within 1 second on average.
-
Scalability:
- The system should be scalable to accommodate a growing number of car insurance policies and client records.
- It should support at least 100 client profiles initially and be able to scale to 1,000 over time.
-
Usability:
- A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
- The CLI and GUI interfaces should be intuitive and user-friendly, allowing agents to perform tasks efficiently.
- The system should provide clear error messages and support for keyboard shortcuts for CLI users.
-
Maintainability:
- The codebase should follow industry best practices and be well-documented to facilitate maintenance and future updates.
-
Interoperability:
- The system should be compatible with various operating systems commonly used by insurance agents.
- It should support importing/exporting data in standard formats for interoperability with other systems.
-
Documentation:
- Comprehensive documentation, including user manuals and technical guides, should be available to assist users and administrators.
- The documentation should be regularly updated to reflect changes and improvements to the system.
Glossary
- User: Car insurance agent using the InsureIQ app
- Client: Buyers / potential buyers that car insurance agent is in contact with, such that it is stored inside InsureIQ
- Client list: List of clients and their personal and policy details in the InsureIQ app
- Address book: Same as client list. Kept in code and some explanation as it is the underlying functionality of InsureIQ
- Mainstream OS: Windows, Linux, Unix, OS-X
- Standard formats: JSON

Appendix: Instructions for manual testing
Given below are instructions to test the application manually.

Launch and shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder.
-
Double-click the jar file.
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
Deleting a person
Deleting a person when persons are being shown
-
Prerequisites: Display persons using the
list
command or other alternative commands such asfind
. At least one person in the list. -
Test case:
delete 1
Expected: First contact is deleted from the displayed list. Details of the deleted contact shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No person is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
,...
(where x is larger than the list size)
Expected: Similar to previous.
Adding a person
-
Adding a client without a policy
-
Prerequisites: All compulsory parameters must be provided.
-
Test case:
add n/Amy Johnson i/951Q p/12345678 e/amy.j@example.com a/123 Main Street t/friends l/SBC9876D
Expected: Robert Green is added to the data file without a policy. Details of the added person are shown in the status message. Timestamp in the status bar as well as the display list is updated. -
Test case:
add n/Robert Green i/234F e/robert.g@example.com a/456 Oak Avenue t/neighbors l/SYZ1234E
Expected: Error details shown in the status message. Timestamp in the status bar is updated. Status bar as well as the display list remains the same. -
Test case:
add n/Amy Johnson i/951Q p/12345678 e/amy.j a/123 Main Street t/friends l/SBC9876D
Expected: No person is added because one of the inputs is not in the correct format. Error details shown in the status. Timestamp in the status bar is updated. Status bar as well as the display list remains the same.
-
-
Adding a client with a policy
-
Prerequisites: All policy parameters must also be provided along with the compulsory non policy parameters.
-
Test case:
add n/Jennifer White i/789M p/98765432 e/jennifer.w@example.com a/789 Pine Street t/colleagues l/SEF5678P c/InsuranceCo pn/456X pi/10-11-2023 pe/09-11-2024
Expected: Details of the added person with policy are shown in the status message. Timestamp in the status bar as well as the display list is updated. -
Test case:
add n/Emily Lim i/345H p/34567890 e/emilylim@example.com a/Blk 345 Jurong t/family c/XYZ Insurance pn/789Z
Expected: Error details shown in the status. Timestamp in the status bar is updated. Status bar as well as the display list remains the same. -
Test case:
add n/Jennifer White i/789M p/98765432 e/jennifer.w@example.com a/789 Pine Street t/colleagues l/SEF5678P c/InsuranceCo pn/456X pi/10-11-2023 pe/09-11-2022
Expected: No person is added to the data file because the policy issue date falls impossibly after the expiry date. Error details shown in the status message. Timestamp in the status bar is updated. Status bar as well as the display list remains the same. -
Test case:
add n/Jennifer White i/789M p/98765432 e/jennifer.w@example.com a/789 Pine Street t/colleagues l/SEF5678P c/InsuranceCo pn/456X pi/10-11-2023 pe/09-11-2024
in a data file where the same person has already been added.
Expected: No person is added because the same person already exists in the data file. Furthermore, even if one compulsory field was different, the policy number cannot be reused. Error details shown in the status message. Timestamp in the status bar is updated. Status bar as well as the display list remains the same.
-
Help command
-
Prerequisites: None
- Test case:
help
Expected: Details of the help page are shown in the status message. - Other test cases that should work include
help
orhelp 2
Expected: Similar to previous
List command
-
Prerequisites: None
- Test case:
list
Expected: Successful listing message is shown in the status message. All the persons added to the program are displayed. - Other test cases that should work include
list
orlist 2
Expected: Similar to previous
Clear command
-
Prerequisites: None.
- Test case:
clear
Expected: Successful clear message is shown in the status message. All clients in the data file are removed. - Other test cases that should work include
clear
orclear 2
Expected: Similar to previous
Editing a person
-
Editing a client without a policy
-
Prerequisites: Display persons using the
list
command or other alternative commands such asfind
. At least one person in the list. The person to be edited is specified using their index number in the shown list. At least one parameter must be specified. If the policy is to be edited successfully, all four parameters must be specified or an error message is shown. -
Test case:
edit 2
Expected: Error details shown in the status message. Timestamp in the status bar is updated. Status bar remains the same. -
Test case:
edit 2 n/Alfred a/Batcave
Expected: Details of the edited person are shown in the status message. Timestamp in the status bar as well as the displayed list are updated. -
Test case:
edit 2 c/Apple
Expected: Error details shown in the status message. Timestamp in the status bar is updated. Status bar remains the same. -
Test case:
edit 2 c/Apple pn/1234 pi/10-10-2003 pe/10-10-2004
Expected: Details of the edited person are shown in the status message. Timestamp in the status bar as well as the displayed list are updated. -
Other incorrect delete commands to try:
edit
,edit x
,...
(where x is larger than the list size)
Expected: Similar to previous. Error details shown in the status message. Timestamp in the status bar is updated. Status bar remains the same.
-
-
Editing a client with a policy
-
Prerequisites: At least one client must be displayed. The person to be edited is specified using their index number in the shown list. At least one parameter must be specified. Not all policy parameters must be specified, but if one of the edits is the same as the respective default value, then the entire policy is deleted.
-
Test case:
edit 2 pn/6969
The person at index 2 is edited with changes toPolicy Number
. Details of the edited person are shown in the status message. Timestamp in the status bar as well as the displayed list are updated. -
Test case:
edit 2 pn/NOPOLICY
Expected: NOPOLICY is the default Policy Number value. The person at index 2 is edited with changes to all four policy parameters to default value such that there isNo Policy Found
. Details of the edited person are shown in the status message. Timestamp in the status bar as well as the displayed list are updated.
-
Finding a person
-
Prerequisites: Display persons using the
list
command or other alternative commands such asfind
. At least one person in the list. At least one parameter must be specified. Using find with default policy parameters will yield no results. -
Test case:
find
Expected: Error details shown in the status message. Timestamp in the status bar is updated. Status bar as well the displayed list remains the same. -
Test case:
find pn/NOPOLICY
Expected: NOPOLICY is the default Policy Number value. Successful find command call is shown in the status message. No clients are displayed. -
Test case:
find n/John
Expected: Successful find command call is shown in the status message. Clients withName
John are displayed.
Sort command
-
Prerequisites: Display persons using the
list
command or other alternative commands such asfind
. At least one person in the list. - Test case:
sort
Expected: Successful sorting message is shown in the status message. Timestamp in the status bar as well as the displayed list are updated. - Other test cases that should work include
sort
orsort 2
Expected: Similar to previous.
Batch Delete Command
-
Prerequisites: Batch Delete works on all clients and not just those that are displayed. One of company or delete month parameters must be specified but not both.
- Test case:
batchdelete dm/12-2019
Expected: Successful batch delete message is shown in the status message. Timestamp in the status bar as well as the displayed list are updated. - Test case:
batchdelete dm/12-2016 c/Apple
Expected: Error details shown in the status message. Timestamp in the status bar is updated. Status bar as well the displayed list remains the same. - Test case:
batchdelete
Expected: Error details shown in the status message. Timestamp in the status bar is updated. Status bar as well the displayed list remains the same.
Remind command
-
Prerequisites: Remind works on all clients and not just those that are displayed. The compulsory parameter must be of type integer and within the specified range.
- Test case:
remind 420
Expected: Successful remind message is shown in the status message. Timestamp in the status bar as well as the displayed list are updated. -
Test case:
remind
Expected: Error details shown in the status message. Timestamp in the status bar is updated. Status bar as well the displayed list remains the same. - Test case:
remind -1
Expected: Error details shown in the status message. Timestamp in the status bar is updated. Status bar as well the displayed list remains the same.
Remark command
-
Prerequisites: Display persons using the
list
command or other alternative commands such asfind
. At least one person in the list. The person to whom we are removing or adding a remark is specified using index. - Test case:
remark 2 r/Contact soon
Expected: Successful remark add message is shown in the status message. Timestamp in the status bar as well as the displayed list are updated. - Test case:
remind 2
orremark 2 r/
Expected: Successful remark add message is shown in the status message. Timestamp in the status bar as well as the displayed list are updated. - Test case:
remark
Expected: Error details shown in the status message. Timestamp in the status bar is updated. Status bar as well the displayed list remains the same.
Exit command
-
Prerequisites: None
- Test case:
exit
Expected: Successful exit message is shown in the status message. The program is then closed. - Other test cases that should work include
exit
orexit 2
Expected: Similar to previous
Appendix: Effort
Achievements of the project
-
Policy
class was added with 4 attributes (company, policy number, issue date, expiry date) -
Person
class was extended to include new attributes (NRIC, licence plate, remark,Policy
) to cater to car insurance agents managing clients - Features added:
batchdelete
,remark
,remind
,sort
- Decided after researching on car insurance and the work of some agents, with more description and justifications found in the main body
- Enhancements to existing features:
-
find
searches by the various fields instead of only name as implemented in AB3 to facilitate car insurance agents’ work - Fields are case-insensitive (e.g. licence plate is stored in capital letters, but users can input small letters) to aid a fast typist
-
- Enhanced testing of features, which led to code coverage increasing from 75% in AB3 to ~80% in InsureIQ
- >8,000 LoCs added
Challenges faced
The challenges mainly arose due to the major extension of the Model
class
- Decision on how to handle the
Policy
details (design considerations mentioned inModel
) - Changes in almost all files to accommodate the newly extended fields, including changes in constructors and implementations to support the nested
Policy
- Changes in UI to present the new fields clearly without making everything seemed to cluttered as they are all text based
- Testing required a lot more cases to handle different cases with different fields
Appendix: Planned Enhancements
Below are the planned enhancements to add in the near future to existing features.
Deleting policy attached to client
- The current implementation of
edit
does not restrict the users to pass in the default values for the Policy fields i.e.!@#NO_COMPANY!@#
forCompany
,NOPOLICY
forPolicyNumber
and01-01-1000
forPolicyDate
- Hence, if any of the default values of the Policy fields is passed in the
edit
command e.g.edit 1 c/!@#NO_COMPANY!@#
oredit 1 pn/NOPOLICY
, it deletes the policy attached to that client at index 1 - We plan to make a separate command (potential name:
policydelete
) to allow users to delete the policy attached to a client, and restrict the user from using the default values in theedit
command

add
command does not restrict the user from using default values for the Policy fields as well.
However, it doesn’t matter since the Policy field in add
is optional. Display clients with expired policy
- The current implementation of
remind
only shows clients with policy expiry dates that are approaching within a certain number of days as specified by the user e.g.remind 30
will show clients with policy expiry dates within the next 30 days,remind 60
will show clients with policy expiry dates within the next 60 days - However, for clients whose policy expiry dates passed the current date,
remind
does not display those clients - Hence, the
remind
command will not be able to accurately display the list of clients as it excludes those clients whose policy expiry dates passed the current date - We plan to make a separate command (potential name:
expiry
) to display the list of clients that has policy expiry dates passed the current date
Retrieving previous successful commands
- The current implementations of all commands is as such:
- If the command fails, the command will be highlighted in red and presents the error message in the result box
- If the command succeed, the result of the command will be shown in the result box and the changes to the client list
- If the command fails, the command will be highlighted in red and presents the error message in the result box
- With each successful command, the command box will be cleared and the result box will show the latest successful command
- Hence, if there are multiple successful commands, the user will have no ability to keep track on what commands he used so far
- We plan to allow the recovery of previously succeeded commands using the up arrow key (Just like in UNIX OS)
Sort should not sort data file
- The current implementation of
sort
not only sorts the client list that is displayed to the user, but also sorts the storage file that stores the client details i.e.[JAR file location]/data/insureiq.json
- Hence, if the user restarts the application again, the client list displayed to the user will be the already sorted client list
- We plan to update the implementation of the
sort
command such that it will only display the sorted client list to the user, but does not sort the client list in the storage file
Support for multiple policies for same client with same vehicle
- The current implementation of
add
andedit
checks for duplicate client by comparing the basic details (Name, Licence Plate, Email, Phone and Address) and if 2 clients have these same fields,add
andedit
will display the error message to reflect that - Essentially,
add
andedit
only the same client with different vehicle to get a different policy - Hence, if the same client with the same vehicle wants to get more than 1 policy, the
add
andedit
commands restricts that - We plan to update the implementation of the
add
andedit
commands such that for the same client with the same vehicle, it will allow different policies attached to it